Editor .NET - Dependent Field Nightmare
Editor .NET - Dependent Field Nightmare
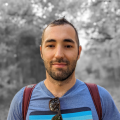
My use-case is very simple, but I have to write some very heavy .NET code to do a "dependent field" validation on my server-side.
The usecase is: If IsVirtual boolean is provided and true, they MUST set VirtualEnvironmentId. If its provided and false, they MUST NOT set VirtualEnvironmentId or provide null/empty.
var response = new Editor(db, "InventoryServer", "Id")
.Validator((editor, action, data) => {
if (action == DtRequest.RequestTypes.EditorEdit ||
action == DtRequest.RequestTypes.EditorCreate)
{
foreach (var entity in data.Data)
{
if (data.Data is null)
{
return null;
}
var entityData = (Dictionary<string, object>)entity.Value;
if (entityData.TryGetValue("InventoryServer", out var inventoryServerDictionary))
{
if (inventoryServerDictionary is Dictionary<string, object> inventoryServer)
{
inventoryServer.TryGetValue("IsVirtual", out var isVirtual);
// TryParse will return false if isVirtual is null or empty.
_ = bool.TryParse(isVirtual?.ToString(), out var isVirtualBoolean);
var virtualEnvironment = inventoryServer.TryGetValue("VirtualEnvironmentId", out var virtualEnvironmentId);
// VirtualEnvironmentId is required when IsVirtual is true.
// virtualEnvironmentId will be an empty string from Editor if it's not set.
if (isVirtualBoolean && string.IsNullOrEmpty(virtualEnvironmentId?.ToString()))
{
return "VirtualEnvironmentId is required when IsVirtual is true";
}
// VirtualEnvironmentId must be sent to us, and must be empty when IsVirtual is false.
if (!isVirtualBoolean && !string.IsNullOrEmpty(virtualEnvironmentId?.ToString()))
{
return "VirtualEnvironmentId must be empty when IsVirtual is false";
}
}
}
}
}
return null;
})
.Field(new Field("InventoryServer.IsVirtual")
.Validator(Validation.Boolean()))
.Field(new Field("InventoryServer.VirtualEnvironmentId")
.SetFormatter(Format.IfEmpty(null))
.Options(new Options()
.Table("VirtualEnvironment")
.Value("Id")
.Label("Name")
)
)
.LeftJoin("VirtualEnvironment", "VirtualEnvironment.Id", "=", "InventoryServer.VirtualEnvironmentId")
.TryCatch(false)
.Debug(true)
.Process(Request)
.Data();
I would like to know, is there an easier way to do this? Is there a way we could get Validator.IsDependentOn()
or something to solve that?
I have to cast and fight my way through many dictionaries and null checks to end up with working code.
Answers
At the moment, there isn't a better way I'm afraid. An
IsDependentOn
method orrequiresField()
or something like that is a nice idea though. I'll look into that - thank you for the suggestion.Allan